Why Stack Overflow Picked Svelte for their Overflow AI Feature And the Website UI
Stack Overflow is a .NET-based monolithic application with JQuery heavily used on the UI along with the Razor templating engine for creating dynamic web pages.
JQuery in today’s UI dev landscape has almost phased out. Modern browsers have evolved their native APIs to the point that websites no longer require JQuery for efficient and consistent DOM manipulation, event handling and AJAX requests.
Modern websites are now powered with frameworks like React, Angular, Vue, Svelte, etc., which offer a declarative component-based approach to building dynamic user interfaces along with their efficient state management.
In contrast, JQuery relies on an imperative style of UI development. The declarative approach facilitates a more structured and modular UI architecture when coding complex UI interfaces.
In the imperative programming paradigm, we have to explicitly specify the steps in code to perform a certain task. The code focuses on explicitly detailing the sequence of operations.
For instance, with code, the developer has to instruct the system to create an element, set its properties, append to the DOM and listen to the events and so on. The dev also has to write the code for managing the element’s state. Well, this is how we write vanilla JavaScript & JQuery code.
In contrast, with declarative frameworks like React, Angular, we may not have to code the low-level details of how an element behaves and its state management. The developer describes what result needs to be achieved without writing step-by-step instructions and the framework manages the elements’ behavior and state.
So, as opposed to the developer writing code to update the DOM, the declarative framework will update the DOM, like React updates the DOM efficiently and consistently by maintaining a virtual DOM. More on this up ahead in the article.
Over the years, after the arrival of NodeJS, Stack Overflow created a lot of tooling leveraging the tech for front-end development, evolving the developer experience. Furthermore, to modernize their UI, they considered frameworks like React, Angular & Svelte.
Component-based Architecture
The new UI needed to have a reusable component-based architecture as opposed to pages being monoliths of repeated UI code across the user interface.
Building page elements in the form of components enables devs to reuse components across different pages of the UI without having to rewrite the entire code, reducing code size and having maintainable code.
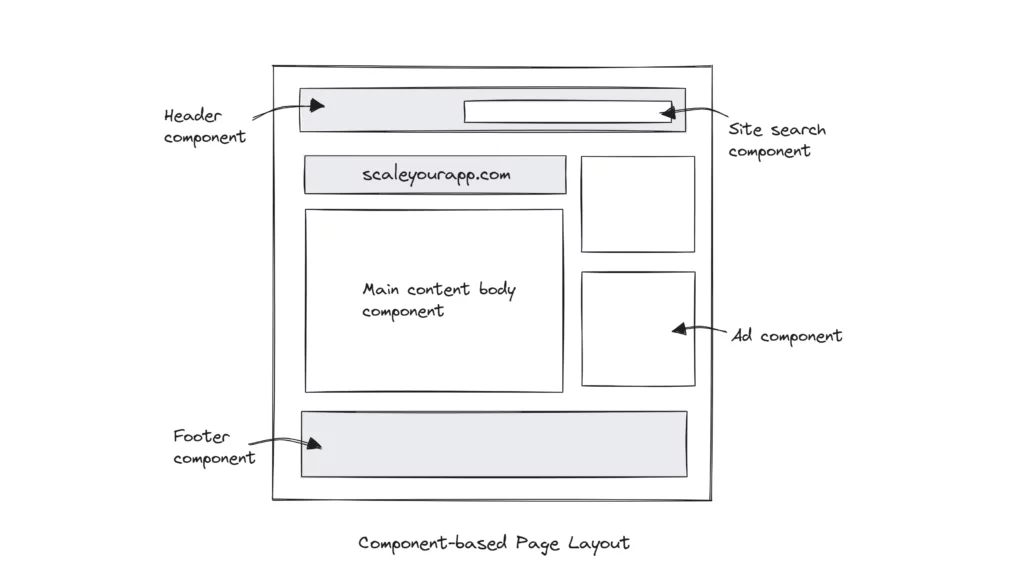
Component-driven development facilitates encapsulation where every component contains the required HTML and JavaScript to build their component and can be unit tested in isolation. Components also encapsulate their internal state and behavior, reducing the odds of unintended interactions between different parts of the system that may cause inconsistencies.
Stack Overflow gave their front-end devs (working on the generative Overflow AI module) a choice to pick whatever tools they wanted to build the new UI and all picked Svelte.
Why Svelte?
Svelte has less of a learning curve than prominent frameworks like React and Angular. The syntax is close to vanilla JavaScript and its simplicity enables devs to do a proof of concept and build a prototype in significantly less time.
Stack Overflow has a lot of full-stack engineers who do a little bit of everything as opposed to focusing on the frontend or the backend. Picking a framework like React or Angular would require significant time investment, whereas Svelte offered a simpler syntax with simplicity; thus, it was chosen.
The second aspect was the performance. SO is accessed across the globe by clients with different internet connectivity and network conditions. Pushing unnecessary UI framework runtime code, which most declarative frameworks leveraging browser runtime environment do, would hit performance, increasing the UI rendering time.
Svelte does not send the unnecessary framework related code to the user’s browser since it does most of the work at compile time. To make this more clear, let’s understand the modern UI development and execution flow.
Modern UI Development And Execution Flow – How Frameworks Render Application Code In the Browsers
Building UI Code
The process of building, compiling and running application UI code varies based on different technologies, frameworks and libraries, though here is a general overview of how modern UI is developed with frameworks like React, Angular, Vue, etc.
Bundling
Once the developer is done writing a component or a UI feature in their IDE, it must first be built to run it, just like the backcode code needs to be built. Why build UI code?
The build process involves bundling, minification, transpiling and optimizing the code for performance. Some popular build tools, such as Webpack, Babel, Parcel, Gulp, Grunt, etc., are leveraged for this.
Code bundling clubs multiple code files into a single optimized file or a set of files to reduce the number of HTTP requests the browser makes to fetch code from the backend. This improves performance starkly.
The bundling tools determine the correct order of the files to be bundled in addition to bundling the dependencies such as stylesheets, images and other assets. The dependencies may also be compressed or converted to a more efficient format before being bundled.
The code is further minified to eliminate whitespaces, comments, renaming variables and such to shorter names etc., to optimize it further.
The code may also be split into smaller bundles to enable them to be loaded on demand. The app UI may not require one big bundle during initial rendering and can fetch additional smaller bundles based on demand. This further helps with performance and improves initial page load time.
Transpiling
Code transpiling involves converting code into a JavaScript version that is supported by a broader range of browsers. For instance, converting ES6 code into earlier versions to be supported on old browsers, converting React JSX code into standard JavaScript, TypeScript to JavaScript, etc.
Both bundling and transpiling happen in the build step of UI development and deployment.
Where does this build process happen?
The build process happens on the developer’s machine and the CI/CD server. Building in the local machine enables the developer to discover any build issues before pushing the code to the remote server. Once everything is okay, the code is pushed to the remote repo and the CI/CD process gets triggered.
The CI/CD server ensures the code is in a deployable state at any time by running builds and tests whenever the code is pushed to the remote repo. In addition, it may also deploy the application to a testing or staging environment for further testing.
Once the code is deployed in production after thorough testing, the browser requests the application UI code from the backend. The code is sent to the client’s browser and modern frameworks whichever the code is written with, React, Angular, Vue or any other, use the browser runtime environment to execute it.
If we take React, for instance, the code execution includes initializing React components, handling state changes and managing virtual DOM.
The virtual DOM is a lightweight replica of the actual DOM. Whenever there is a state change in a React component, it computes the minimal set of changes that need to be made to update the DOM. It then updates the virtual DOM and then those changes get applied to the actual DOM.
From a performance standpoint, DOM manipulations are expensive operations. Thus, React leverages the virtual DOM to perform all manipulations before committing the changes to the real DOM. Also, with virtual DOM, multiple updates can be clubbed together and applied to the actual DOM in one operation, thus improving performance.
Sending the Framework Runtime Library Code to the Browser
To run the code in the browser efficiently, frameworks include the runtime library code along with the core UI code in the browser. The additional runtime library code manages components, states and other framework-specific functionality.
Now, this runtime library code has some performance costs since it is additional code pushed to the client to run the core UI code. This is where Svelte differs.
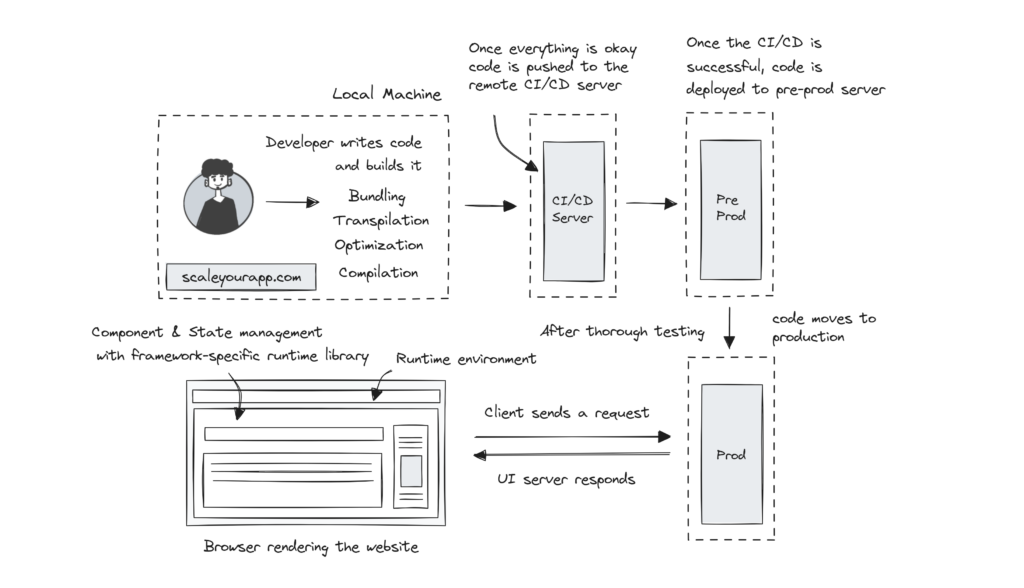
Svelte Does the Heavy Lifting at Compile-time as Opposed to Runtime
Svelte is referred to as more of a compiler than a framework. It takes the compiler-based approach to build web applications. As opposed to leveraging the browser runtime to do most of the work, Svelte does most of the work during compilation (during the build process) in the developer’s machine. It does not push any framework-specific runtime library code to the client’s browser, thus performing better.
It also doesn’t rely on a virtual DOM. It leverages a concept called Reactive declarations that automatically update the DOM when the underlying data changes. Svelte compiler, during code compilation, figures the reactive parts of the code and generates the necessary logic that would update the DOM efficiently in the browser.
The absence of a virtual DOM in Svelte reduces the runtime overhead associated with maintaining and updating a separate in-memory representation of the DOM.
Though Svelte does a significant amount of work during compile time, tracking the state changes still happens at runtime in the browser. When the user interacts with the application and performs state changes, the Svelte code contains the necessary logic, generated at compile time, to handle state changes and update the DOM accordingly.
Svelte at Stack Overflow
SO Engineering did a POC (Proof Of Concept) to see how introducing Svelte would impact the user metric and realized it was better compared to React.
The existing SO UI is rendered on the server side with Razor templating engine that allows faster loading times on the client since the content is rendered on the server and the browser has to do comparatively less work displaying the UI. The idea is to deliver the UI in the quickest amount of time to the client.
In server-side rendering, the server takes care of generating HTML content and this pre-rendered HTML is sent to the browser, which reduces the need for extensive client-side processing to display the UI.
The browser, upon receiving the HTML, can start rendering the page immediately, which results in faster initial page loads. However, server-side rendering has tradeoffs as well. It doesn’t work that great for dynamic web pages where sections of a web page are continuously getting updated. In this scenario, client-side rendering is more performant.
Certain parts of the Stack Overflow website are continually evolving and need interactivity and this required a component-based architecture implementation with Svelte client-side rendering.
The evolving website UI components, along with the new OverflowAI UI, are being built with Svelte. In the new design, independent components are easily testable, loosely coupled, making the UI more maintainable and flexible.
They borrowed a concept called “interactive islands” from another popular UI framework called Astro.
“Astro Island” refers to an interactive UI component on an otherwise static HTML page. Multiple islands can exist on a page, and an island always renders in isolation.
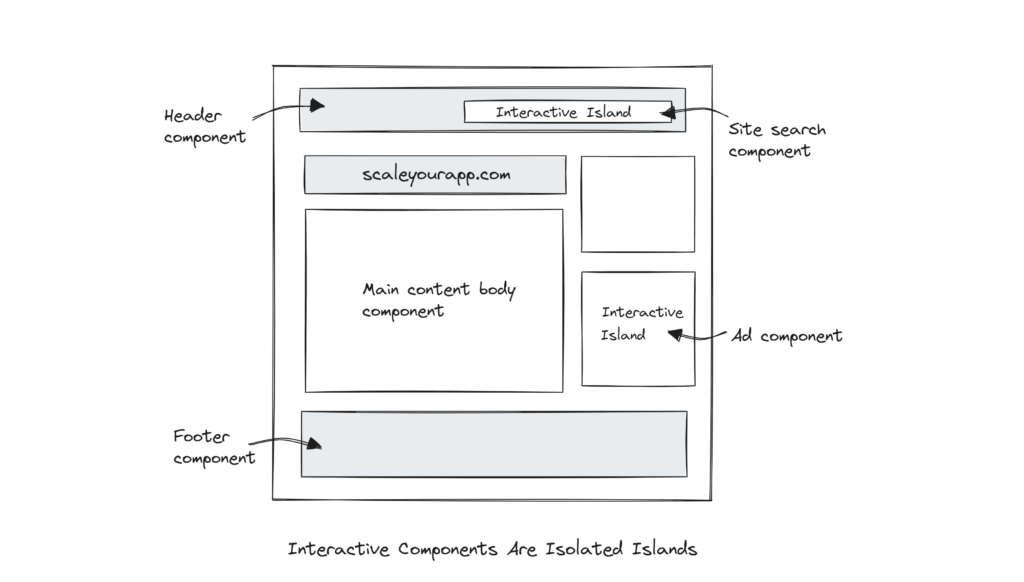
The new islands in the SO UI are developed with Svelte with client-side rendering. Engineers build these interactive islands in isolation, test them, and plug them wherever required on the website.
Information source: Why Stack Overflow is embracing Svelte
If you wish to delve deeper into the backend architecture of large-scale services, with discussions on how large-scale services are designed, including discussions on fundamental concepts like client and server-side rendering, scalability, high availability, message queues, data streaming and more, check out the Zero to Mastering Software Architecture learning path, I’ve authored.
It gives you a structured learning experience, taking you right from having no knowledge on the domain to making you a pro in designing web-scale distributed systems like YouTube, Netflix, ESPN and the like.
Distributed Systems
For a complete list of similar articles on distributed systems and real-world architectures, here you go
If you found the content helpful, consider sharing it with your network for more reach. I am Shivang. Here is my X and LinkedIn profile. Feel free to send me a message. I’ll see you in the next post. Until then, Cheers!
If you wish to receive similar posts along with subscriber-only content in your inbox, consider subscribing to my newsletter.
Shivang
Related posts
Zero to Software Architecture Proficiency learning path - Starting from zero to designing web-scale distributed services. Check it out.
Master system design for your interviews. Check out this blog post written by me.
Zero to Software Architecture Proficiency is a learning path authored by me comprising a series of three courses for software developers, aspiring architects, product managers/owners, engineering managers, IT consultants and anyone looking to get a firm grasp on software architecture, application deployment infrastructure and distributed systems design starting right from zero. Check it out.
Recent Posts
- System Design Case Study #5: In-Memory Storage & In-Memory Databases – Storing Application Data In-Memory To Achieve Sub-Second Response Latency
- System Design Case Study #4: How WalkMe Engineering Scaled their Stateful Service Leveraging Pub-Sub Mechanism
- Why Stack Overflow Picked Svelte for their Overflow AI Feature And the Website UI
- A Discussion on Stateless & Stateful Services (Managing User State on the Backend)
- System Design Case Study #3: How Discord Scaled Their Member Update Feature Benchmarking Different Data Structures
CodeCrafters lets you build tools like Redis, Docker, Git and more from the bare bones. With their hands-on courses, you not only gain an in-depth understanding of distributed systems and advanced system design concepts but can also compare your project with the community and then finally navigate the official source code to see how it’s done.
Get 40% off with this link. (Affiliate)
Follow Me On Social Media